Authentication
Control who can access your endpoints by using authentication.
By default Sheety has no authentication enabled on your API as this is usually suitable for most use cases (as long as the data isn’t sensitive!). Though any methods that edit your sheet (such as POST) are disabled by default.
Sheety supports two types of authentication: Basic auth, and Bearer auth. Both work in similar ways.
Basic Auth
With Basic Auth, a request contains a header field called Authorization
, with the value set to the username and password encoded as base64. You set the username and password inside of Sheety.
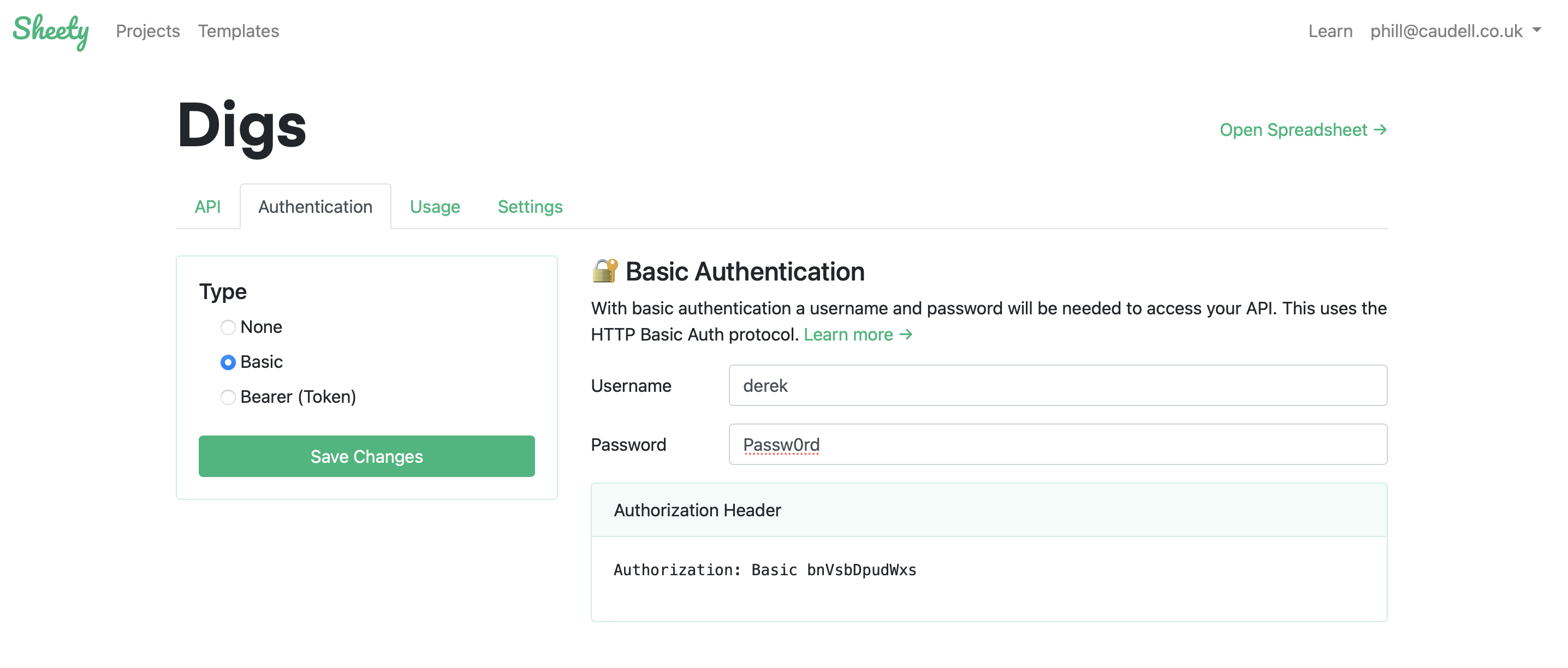
💡 Sheety Tip: Sheety can generate the Authorization header value for you. Find it inside Authentication settings.
Here’s how this looks using Javascript:
var headers = new Headers();
headers.append("Authorization", "Basic bnVsbDpudWxs");
fetch(url, {
method: "GET",
headers: headers
})
.then(response => response.json())
.then(json => {
// Do something with your data
console.log(json);
});
Bearer auth
Bearer authentication works exactly the same as Basic auth, though instead of username and password encoded as base64, it’s simply a token (or secret) of your choosing.